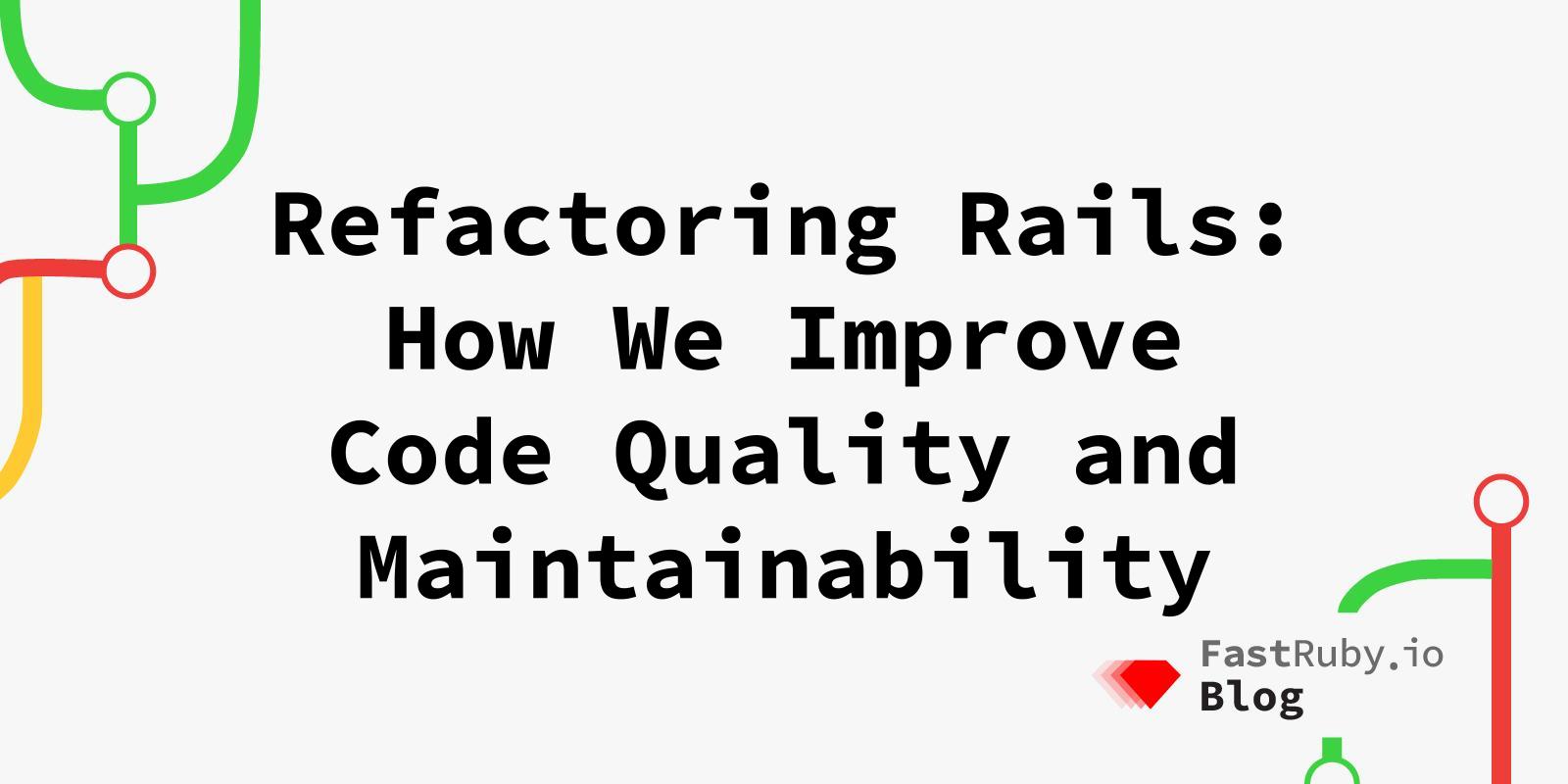
Refactoring Rails: How We Improve Code Quality and Maintainability
In this post, we share our approach to refactoring Rails applications, focusing on improving code maintainability, reducing technical debt, and ensuring scalability. Discover the tools, techniques, and strategies we use to transform complex codebases into cleaner, more efficient systems.
1. Introduction
Refactoring is an essential part of maintaining any Rails application. Over time, as applications evolve and grow, the codebase can become harder to manage, introducing technical debt and slowing down development.
Refactoring helps us address these challenges by improving maintainability and making it possible for smaller teams to effectively work on the codebase. Clear, well-structured code isn’t just easier to read and modify, it also enables developers to quickly identify the relevant components when a change is required. Most importantly, it reduces the risks associated with making modifications, ensuring that enhancements or bug fixes don’t unintentionally introduce new issues.
We approach refactoring with these goals in mind, focusing on creating a codebase that’s both resilient and ready to support future growth.
2. Understanding the Problem
Before diving into any refactoring effort, it’s essential to understand the bigger picture. We start by aligning our work with the client’s business goals. Refactoring is not just about cleaner code, it’s about creating a codebase that supports the company’s objectives, whether that’s faster feature delivery, improved performance, or scaling the application to handle increased demand. By aligning with these goals, we ensure that every change we make delivers real value.
Next, we take time to thoroughly examine the codebase using both quantitative and qualitative methods . A comprehensive analysis gives us insight into the health of the codebase, from its structure to its performance under real-world conditions. We also make it a point to discuss past refactoring efforts with the client. Learning from these experiences is crucial to avoid repeating mistakes and to build on strategies that have worked well. Clients often provide invaluable insights by pointing out pain points and bottlenecks they’ve encountered. We use this input to guide and enhance our analysis.
To identify areas that need improvement, we rely on both static and dynamic analysis tools:
- Dynamic Analysis: These tools evaluate the code while it’s running.
- Exception tracking tools like Sentry or Bugsnag identify and help fix errors.
- Application performance monitoring tools like New Relic or Scout pinpoint performance bottlenecks.
- Coverband measures code usage in production, highlighting parts of the application that are unused.
- Static Analysis: These tools analyze the code without executing it, uncovering
issues like code smells, complexity, and duplication.
- Reek : Detects code smells.
- RuboCop : Analyzes and formats Ruby code.
- Skunk : Calculates a cost metric for each file to identify areas needing attention.
- RubyCritic : Provides a quality report by wrapping tools like Reek, Flay, and Flog.
- Mutant : Highlights untested areas of the codebase using mutation testing.
- SimpleCov : Offers insights into test coverage.
The combination of these tools and techniques ensures a comprehensive understanding of the codebase, allowing us to create a tailored refactoring plan that prioritizes high-impact changes while minimizing risk.
3. Our Approach to Refactoring
Refactoring is a deliberate, methodical process. To ensure success, we break it down into clearly defined steps that help us stay focused on delivering meaningful improvements while minimizing risk. Here’s how we approach it:
3.1 Drafting a Refactoring Plan
Every successful refactoring begins with a well-thought-out plan. Based on our earlier analysis, we identify a specific area of the codebase that needs attention, targeting areas that align with the client’s goals and our refactoring criteria.
- Collaboration: At least two engineers review the code, identifying opportunities for improvement.
- Refactoring Criteria:
- Addressing files in the top-right quadrant of RubyCritic (high complexity and high churn ).
- Prioritizing files in critical paths.
- Investigating recurring exceptions identified by the clients exception tracking tool, as they often indicate deeper issues requiring refactoring or enhanced test coverage.
The findings are consolidated into a comprehensive refactoring plan, which outlines:
- Goals to increase test coverage.
- Plans to remove unused or redundant code.
- Specific design patterns or strategies to be applied during the refactoring.
Before implementation, we share the plan with the client for feedback and approval. Any subsequent changes to the plan are documented and discussed to ensure alignment with the client’s expectations.
3.2 Improving Test Coverage
Test coverage is a cornerstone of safe refactoring. We evaluate the codebase using tools like SimpleCov and Skunk to identify areas with low coverage or high complexity.
- Key Considerations:
- We identify files with high churn (frequently updated files) and high complexity, as these are critical to application stability.
- Data from Coverband helps pinpoint unused code, which we can safely remove to reduce clutter.
- By analyzing patterns in high-cost files using Skunk, we prioritize testing key areas.
We address test coverage gaps as a separate task before refactoring. This ensures that we have a robust safety net to catch regressions or unexpected behavior.
3.3 Executing the Refactoring Plan
With a clear plan in place, we proceed with refactoring in small, focused steps. This methodical approach allows us to address specific issues within the codebase while maintaining stability and predictability.
Our refactoring process is not limited to but includes:
- Separating public and private methods: Public and private methods are clearly distinguished within each class, making it easier to understand the class’s intended use and internal workings.
- Adding explicit behaviors to classes: We prioritize clarity by adding explicit behaviors to classes and reserving implicit logic only for cases where it enhances readability.
- Favoring composition over inheritance: This approach reduces tight coupling and increases flexibility by encapsulating functionality in separate, easily testable components.
- Using service objects instead of callbacks: Small, focused service objects replace implicit callbacks, resulting in clearer, easier-to-maintain code.
- Encapsulating behaviors in classes: Instead of relying on implicit modules or concerns, we move functionality into dedicated classes. This approach improves predictability and testability.
- Streamlining controllers: Controllers are refined to focus on the standard seven RESTful actions. Any custom actions are either extracted into new controllers and routes or restructured into service objects to adhere to the single-responsibility principle.
- Consolidating repeated code: Redundant code is consolidated, and logic that doesn’t belong in a specific file is moved to its appropriate location.
- Improving naming conventions: Meaningful and descriptive names are used for variables, methods, and classes to enhance clarity and maintainability.
- Eliminating code smells: We use insights from Reek to identify and address code smells. This ensures the codebase is sufficiently tidied up, making it easier to understand and maintain in the long term.
By focusing on these targeted improvements, we aim to create a cleaner, more maintainable codebase that aligns with both best practices and the client’s goals.
3.4 Review and Test
Each refactoring step is reviewed and tested independently to catch issues early:
- Small Pull Requests: We keep PRs small, focusing on specific changes to make reviews manageable.
- Automated Testing: Tests verify that no regressions are introduced.
- Manual Testing: Ensures the application behaves as expected from a user perspective.
- Code Reviews: Conducted by senior engineers to maintain high standards.
For larger refactoring efforts, we use a long-running Git branch to isolate the work. This branch is regularly updated with the main branch to stay current and is used to merge incremental changes. Once all steps are complete, the QA team reviews the long-running branch for regressions before merging it into the main branch.
By following this structured approach, we ensure that our refactoring efforts deliver measurable improvements without disrupting ongoing development.
4. Common Challenges and How We Overcome Them
Refactoring projects often come with unique challenges. Over time, we’ve developed strategies to address these challenges effectively, ensuring that our efforts deliver meaningful results while aligning with the client’s needs.
4.1 Clear Communication with the Client
Effective communication is vital to ensure our refactoring efforts align with the client’s goals and expectations.
- We involve the client in the planning process from the outset, providing opportunities to share their insights and address concerns.
- Regular updates throughout the project allow us to confirm alignment and adjust our approach as needed.
- By maintaining transparency, we build trust and ensure that the client feels confident in the direction of the refactor.
4.2 Quick Feedback Loops
A short feedback loop helps us refine our approach and address issues promptly.
- Small, Focused Pull Requests: Breaking down the work into manageable chunks makes it easier for the client to review and provide feedback.
- Regular Check-ins: We prioritize frequent communication to ensure we’re staying on track and can course-correct as necessary.
4.3 Navigating Undocumented Legacy Code
Dealing with legacy code without documentation can be challenging, but we employ several strategies to handle it effectively:
- Identifying Unused Code: Tools like Coverband and Debride help us identify code that’s no longer in use, which we can safely remove to simplify the codebase.
- Backward-Compatible Changes: When making significant changes, such as restructuring controllers, we implement fallback solutions like redirect routes to maintain compatibility.
- Gradual Deprecation: Unused code is deprecated incrementally, with plans to remove it entirely after a few release cycles. This approach minimizes disruption while encouraging adoption of the updated structure.
4.4 Managing Limited QA Resources
Quality assurance is crucial, but limited QA resources can create bottlenecks.
- We create long-running branches to consolidate refactoring efforts incrementally. This allows smaller PRs to be reviewed independently, saving QA resources for the final consolidated branch.
- By organizing work into smaller, reviewable increments, we streamline the QA process while maintaining high standards.
4.5 Balancing Effort with Value
To ensure the refactoring effort is efficient and impactful, we focus on delivering maximum value.
- Prioritizing High-Churn Areas: We target areas with high churn and complexity, as these are often critical to stability and performance.
- Essential vs. Optional Work: We categorize refactoring tasks into essential and optional efforts, giving the client the flexibility to prioritize based on their goals and constraints.
By addressing these common challenges head-on, we ensure our refactoring process is effective, efficient, and aligned with the client’s objectives. This approach helps us deliver high-quality results while navigating the complexities of legacy systems and dynamic requirements.
5. Conclusion
Refactoring a Rails application can be challenging, but with a structured approach and the right tools, it’s possible to significantly improve maintainability and reduce complexity. By aligning refactoring efforts with business goals and addressing common challenges thoughtfully, we can create a codebase that’s easier to work with and better equipped to support future growth.
Need help with your Rails application? Talk to us today!